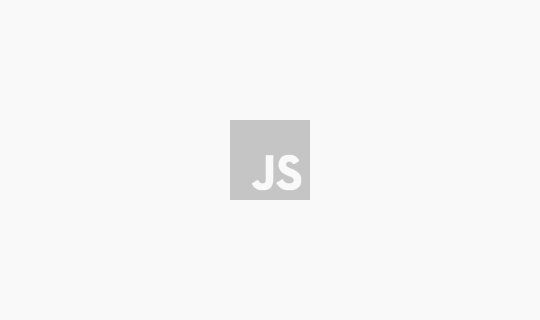
[소소한 개발 일지] WeakRef의 약한 참조와 메모리 관리
2025-03-13
간단하게 TypeScript + React + Webpack 구성하기
2019-09-04
Explanation
오늘은 간단하게 타입스크립트 + 리액트 + 웹팩 구성을 만들어 보려 합니다.
예제 코드는 깃헙 저장소에 올려놓았습니다.
https://github.com/falsy/blog-post-example/tree/master/typescript-react-webpack-starter-kit
하나하나 설치하려면 힘드니까, 아래와 같은 package.json 파일을 만들고 한번에 install 할게요.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
//package.json { "name": "typescript-react-webpack-starter-kit", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "start": "webpack-dev-server --mode development", "build": "webpack --mode development" }, "author": "falsy", "license": "WTFPL", "devDependencies": { "@types/react": "^16.9.2", // 타입스크립트로 react를 사용하기 위한 패키지 "@types/react-dom": "^16.9.0", // 타입스크립트로 react-dom을 사용하기 위한 패키지 "html-webpack-plugin": "^3.2.0", // html에 번들을 넣어주는 웹팩 플러그인 "react": "^16.9.0", // 리엑트 "react-dom": "^16.9.0", // 리엑트 돔 "source-map-loader": "^0.2.4", // 소스맵 로더 "ts-loader": "^6.0.4", // 타입스크립트 로더 "typescript": "^3.6.2", // 타입스크립트 "webpack": "^4.30.0", // 웹팩 "webpack-cli": "^3.3.1", // 웹팩 CLI "webpack-dev-server": "^3.3.1" // 웹팩 개발 서버 }, "dependencies": {} } |
그리고 설치
1 |
$ npm install |
우선 최종적으로 파일 구조는 아래와 같이 할꺼에요.
1 2 3 4 5 6 7 8 |
/node_modules ... /src App.tsx index.html index.tsx tsconfig.json webpack.config.js |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
// webpack.config.js const HTMLWeebPackPlugin = require('html-webpack-plugin'); // 아까 설치한 플러그인이죠? html 문서에 자동으로 번들파일을 추가해줍니다. const path = require('path'); module.exports = { entry: './src/index.tsx', // 처음 시작할 파일을 지정해줍니다. 지정하지 않으면 './src/index.js'가 기본 값이기 때문에 적어줘야 해요 module: { rules: [ { test: /\.tsx?$/, // .tsx 확장자로 끝나는 파일들을 use: 'ts-loader', // ts-loader 가 트랜스파일 해줍니다. exclude: /node_modules/ // node_modules 디렉토리에 있는 파일들이 제외하고 }, { enforce: "pre", test: /\.js$/, loader: "source-map-loader" } ] }, resolve: { extensions: [ '.tsx', '.ts', '.js' ] }, output: { filename: 'bundle.js', // build시 만들어질 파일 번들 파일 이름 path: path.resolve(__dirname, 'dist') // 그리고 경로 입니다. }, plugins: [ new HTMLWeebPackPlugin({ template: './src/index.html', filename: './index.html' }) // './src/index.html' 경로의 html 파일에 번들 파일을 넣어줍니다. ] }; |
1 2 3 4 5 6 7 8 9 10 11 |
// tsconfig.json { "compilerOptions": { "outDir": "./dist/", // 아웃풋 될 경로 "noImplicitAny": true, // 음.. 암시적 any 타입의 표현식 및 선언에서 오류를 발생시킬지? "module": "es6", "target": "es5", "jsx": "react", "allowJs": true // .js 파일도 함께 컴파일 할지 여부 } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
// src/App.tsx import * as React from 'react'; import TodoItem from './components/TodoItem'; interface Hello { compiler: string; framework: string; } export class App extends React.Component<Hello, {}> { render() { return ( <h1> Hello from {this.props.compiler} and {this.props.framework}! </h1> ); } } |
1 2 3 4 5 6 7 |
// src/index.tsx import * as React from 'react'; import * as ReactDOM from 'react-dom'; import { App } from './App'; ReactDOM.render(<App compiler="TypeScript" framework="React" />, document.getElementById('app')); |
1 2 3 4 5 6 7 8 9 10 11 |
<!-- src/index.html --> <!DOCTYPE html> <html lang="ko"> <head> <meta charset="UTF-8" /> <title>Typescript - React</title> </head> <body> <div id='app'></div> </body> </html> |
1 |
$ npm start |
https://www.typescriptlang.org/docs/handbook/react-&-webpack.html
https://typescript-kr.github.io/
https://www.kidolog.com/post/5c3dcd1dae087873de985fd3
https://vomvoru.github.io/blog/tsconfig-compiler-options-kr/