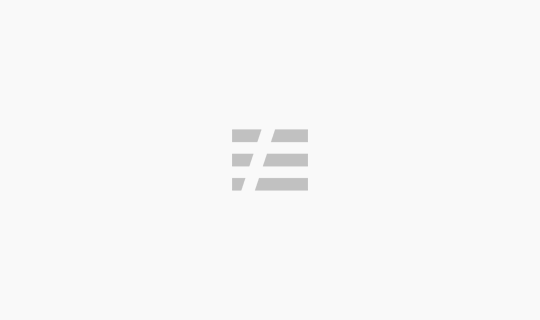
[새로운 버전] Serverless Framework를 사용하여 NextJS 프로젝트를 AWS Lambda를 통해 배포하기
크롬 확장 프로그램 만들기, GCM 알림 받기
2018-04-07
Explanation
간단하게 크롬 확장프로그램을 개발하는 방법부터 GCM(Google Cloud Messaging)을 이용하여 웹 알림(Notification)을 사용하는 방법에 대하여 적어보려 합니다. (웹 알림을 전송을 위한 API는 간단하게 노드를 통해 개발, 테스트합니다.)
GCM은 FCM(Firebase Cloud Messaging)으로 새로운 버전이 나왔으며 FCM은 GCM의 인프라와 새로운 기능들을 담고 있습니다.
우선 빈 디렉토리를 만들고 ‘manifest.json’과 ‘index.html’ 파일을 만듭니다. (저는 extensions-cgs 라는 이름으로 디렉토리를 만들었습니다.)
1 2 3 4 5 6 7 8 9 10 |
// extensions-cgs/manifest.json { "name": "CGS-Helloword", "description" : "helloword", "version": "1.0", "browser_action": { "default_popup": "index.html" }, "manifest_version": 2 } |
1 2 3 4 5 6 7 8 9 10 11 |
<!-- extensions-cgs/index.html --> <!DOCTYPE html> <html> <head> <title>Cheolguso Notification</title> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> </head> <body> hello world </body> </html> |
크롬 브라우저에서 주소창에 ‘chrome://extensions/‘ 로 이동하여 ‘압축해제된 확장 프로그램을 로드합니다.’ 를 선택하신 후 ‘extensions-cgs’ 디렉토리를 불러오면 끝 입니다.
브라우저 오른쪽 위에 새로 등락한 확장프로그램을 선택하시면 ‘hello world’ 문구를 확인 할 수 있습니다.
manifest의 다양한 속성에 관한 정보는 크롬 디벨롭 사이트를 통해서 확인 하실 수 있습니다.
https://developer.chrome.com/extensions/manifest
Firebase console 페이지로 이동하여 로그인 후 프로젝트를 생성하고 프로젝트에 접속합니다.
왼쪽 메뉴의 ‘Project Overview’ 옆의 톱니바퀴(settings)를 선택 -> ‘프로젝트 설정’ 선택 -> ‘클라우드 메시징’ 선택
이곳에서 확인하실 수 있는 ‘서버키’, ‘발신자 ID’를 사용합니다.
1. 확장프로그램에서 GCM을 통해 메세지를 받을 수 있도록 설정합니다.
2. 확장프로그램에서 GCM에 등록하고 등록키 값을 확인합니다.
3. 해당 확장프로그램으로 알림을 전송할 수 있는 API를 만듭니다.
가로세로 각각 128px의 이미지를 하나 만들어 추가하였습니다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
// manifest.json { "name": "CGS-Notifications", "description" : "Notifications", "version": "1.0", "browser_action": { "default_popup": "index.html", "default_icon": "icon-128.png" }, "manifest_version": 2, "permissions": ["gcm", "notifications"], // "gcm", "notification" 권한을 사용 "background": { "scripts": ["background.js"], // 백그라운드로 실행할 스크립트 파일 "persistent": false } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
<!-- index.html --> <!DOCTYPE html> <html> <head> <title>Cheolguso Notification</title> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <style> body {width: 500px; height: 50px;} </style> </head> <body> <script src="./script.js"></script> </body> </html> |
1 2 3 4 5 |
// script.js const senderIds = ['123456789..']; // Firebase console에서 확인한 발신자 ID chrome.gcm.register(senderIds, function(registrationId) { // gcm 등록 document.write('<p>RegistrationId : ' + registrationId + '</p>'); // 등록 아이디 }); |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
// background.js function getNotificationId() { var id = Math.floor(Math.random() * 9007199254740992) + 1; return id.toString(); } function messageReceived(message) { var messageString = ""; for (var key in message.data) { if (messageString != "") messageString += ", " messageString += key + ":" + message.data[key]; } //getNotificationId는 같은 값이면 알림이 출력되지 않기 때문에 매번 새로운 아이디 부여 chrome.notifications.create(getNotificationId(), { title: 'Notisave', iconUrl: 'icon-128.png', type: 'basic', message: messageString, }, function() {}); } chrome.gcm.onMessage.addListener(messageReceived); |
최종적으로 파일 목록은 아래와 같습니다
1 2 3 4 5 6 |
/extensions-cgs background.js icon-128.png index.html manifest.json script.js |
모두 수정하신 후애 ‘chrome://extensions/‘에서 다시 ‘압축해제된 확장 프로그램을 로드합니다.’를 통해 업로드합니다.
이제 만들어진 확장프로그램을 클릭하면 등록된 레지스트아이디를 출력하는 확장프로그램이 되었습니다.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
const express = require('express'); const app = express(); const bodyParser = require('body-parser'); const request = require('request'); app.use(bodyParser.json()); app.post('/push', (req, res) => { const serverKey = 'AAAAL8nArq...'; // Firebase console에서 확인한 서버키 값 const auth = 'key=' + serverKey; const key = req.body.key; const text = req.body.text; const registrationId = req.body.registrationId; // 추가한 구글 확장프로그램을 선택했을때 출력됐던 등록키 값 request.post({ url: 'https://android.googleapis.com/gcm/send', headers: { Authorization: auth }, form: { registration_id : registrationId, ['data.'+key] : text }}, function(err, respon, body) { res.send(); }); }); app.listen(3000, function () { console.log('Example app listening on port 3000!'); }); |
이제 ‘Postman‘ 같은 툴을 사용해서 ‘localhost:3000/push’로 body에 json형태로 ‘registrationId’, ‘key’, ‘text’ 값을 전송하면 알림이 전송됩니다.
끄읕.
참고 했던 문서의 링크를 잊어버려서.. 차후에 찾아서 추가하겠습니다.