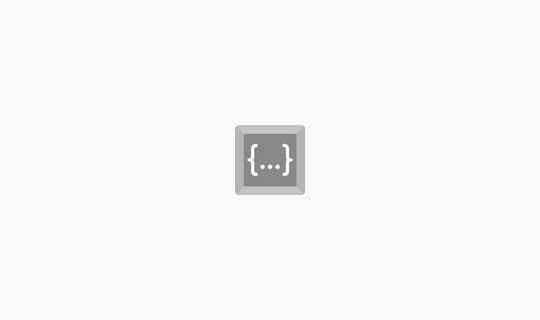
Xcode에서 Codeium 사용하기
2023-12-31
Enzyme + Jest + React + Webpack 테스트 코드 작성하기
2019-09-10
Explanation
오늘은 간단하게 Webpack과 Enzyme, Jest를 사용해서 React 컴퍼넌트를 테스트하는 환경? 구성? 을 만들어 보려고 합니다.
어디까지나 아주 간략한 시작점이고 이후는 Jest 공홈이나 구글 검색을 통해 하나씩 추가해 나가면 좋을거 같아요.
역시 코드는 깃허브 저장소에 올려놓았습니다~
https://github.com/falsy/blog-post-example/tree/master/enzyme-jest-react
역시.. 하나하나 인스톨하려면 힘드니까, 원하시는 디렉토리에 아래의 package.json 파일을 만들고 한방에 install 할게요.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
//package.json { "name": "enzyme-jest-react", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "start": "webpack-dev-server --mode development", "test": "jest" }, "repository": { "type": "git", "url": "git+https://github.com/falsy/enzyme-jest-react.git" }, "author": "falsy.me", "license": "WTFPL", "bugs": { "url": "https://github.com/falsy/enzyme-jest-react/issues" }, "homepage": "https://github.com/falsy/enzyme-jest-react#readme", "dependencies": { "react": "^16.9.0", "react-dom": "^16.9.0" }, "devDependencies": { "@babel/core": "^7.5.5", "@babel/preset-env": "^7.5.5", "@babel/preset-react": "^7.0.0", "babel-jest": "^24.9.0", "babel-loader": "^8.0.6", "css-loader": "^3.2.0", "enzyme": "^3.10.0", "enzyme-adapter-react-16": "^1.14.0", "html-loader": "^0.5.5", "html-webpack-plugin": "^3.2.0", "jest": "^24.9.0", "node-sass": "^4.12.0", "sass": "^1.3.0", "sass-loader": "^8.0.0", "style-loader": "^1.0.0", "webpack": "^4.39.3", "webpack-cli": "^3.3.7", "webpack-dev-server": "^3.8.0" } } |
그리고 설치
1 |
$ npm install |
그리고 최종적으로 파일 구조는 아래와 같이 할꺼에요.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
/src /__TEST__ sample.spec.js /components Sample.js /styles sample.scss index.html index.js .babelrc jest.config.js packgage.json webpack.config.js |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
// webpack.config.js const { resolve } = require('path'); const HTMLWeebPackPlugin = require('html-webpack-plugin'); module.exports = { output: { path: resolve(__dirname, 'src/dist'), publicPath: '/' }, module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader' } }, { test: /\.html$/, use: [ { loader: 'html-loader', options: { minimize: true } } ] }, { test: /.scss$/, use: [ 'style-loader', { loader: 'css-loader', options: { modules: true } }, 'sass-loader' ] } ] }, plugins: [ new HTMLWeebPackPlugin({ template: resolve(__dirname, 'src/index.html'), filename: './index.html' }) ] }; |
1 2 3 4 5 6 |
// jest.config.js module.exports = { moduleNameMapper: { '^.+\\.(css|less|scss)$': 'babel-jest' } }; |
1 2 3 4 |
// .babelrc { "presets": ["@babel/preset-env", "@babel/preset-react"] } |
1 2 3 4 5 6 7 8 9 10 11 |
<!-- src/index.html --> <!DOCTYPE html> <html lang="ko"> <head> <meta charset="UTF-8" /> <title>Sample</title> </head> <body> <div id='app'></div> </body> </html> |
1 2 3 4 5 6 7 8 9 10 11 12 13 |
// component/Sample.js import React from 'react'; import { statusPage, pageTitle } from '../styles/sample.scss'; const Sample = () => { return ( <section className={statusPage}> <h1 className={pageTitle}>SAMPLE PAGE</h1> </section> ); }; export default Sample; |
1 2 3 4 5 6 7 |
// styles/sample.scss .statusPage { padding: 40px; } .pageTitle { font-size: 20px; } |
1 2 3 4 5 6 7 8 9 10 11 |
// __TEST__/sample.spec.js import React from 'react'; import Enzyme, { shallow } from 'enzyme'; import Adapter from 'enzyme-adapter-react-16'; import Sample from '../components/Sample'; Enzyme.configure({ adapter: new Adapter() }); test('check status component redner', () => { const component = shallow(<Sample />); expect(component.text()).toEqual('SAMPLE PAGE'); }); |
1 2 |
// 실행 $ npm start |
1 2 |
// 테스트 $ npm test |
음… 설명은 없고 온통 코드만 적었네요…
시간이 너무 늦고, 피곤해서.. 설명은.. 다음 기회에..